Petit Lynx
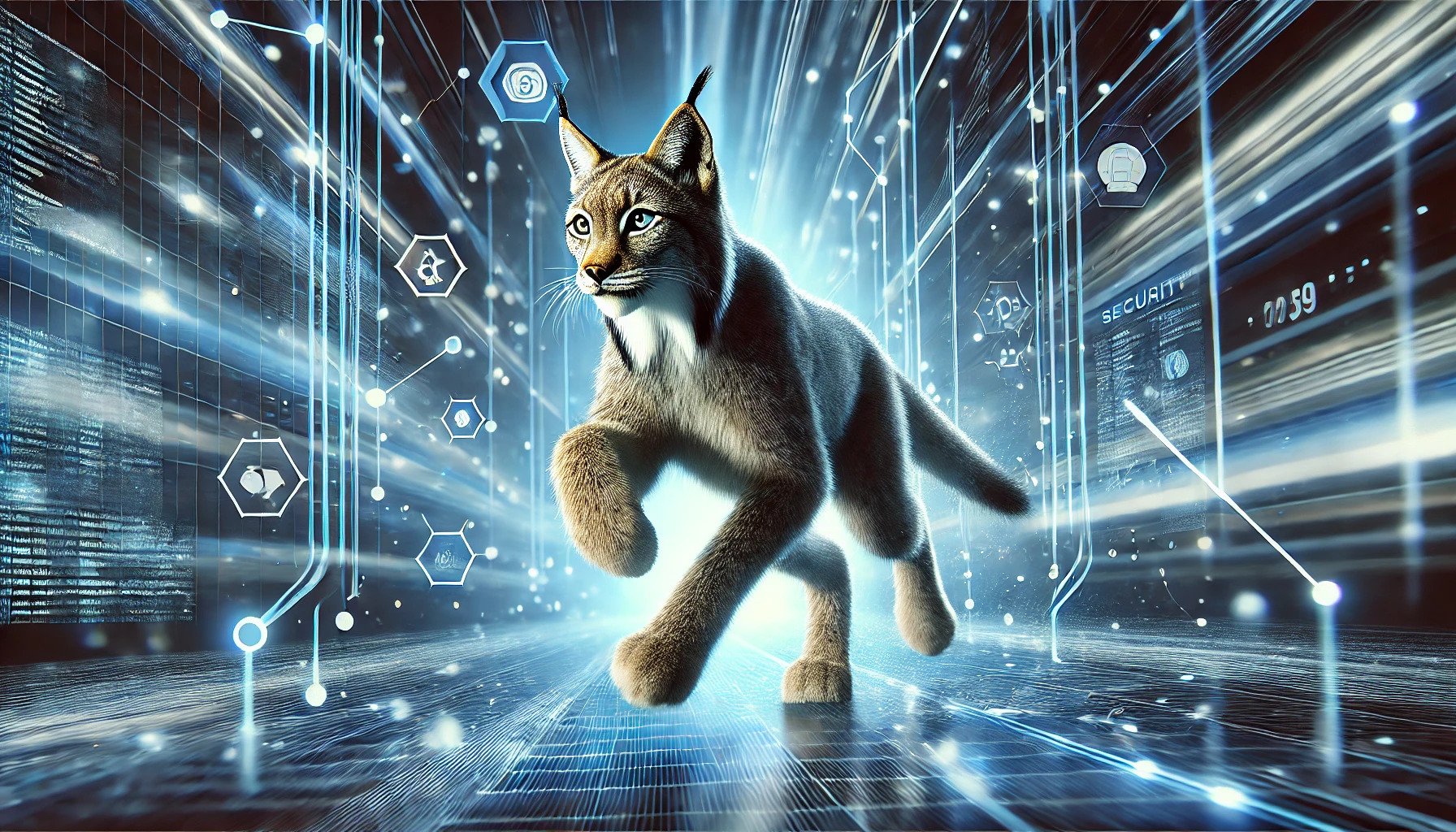
Lynx is a instruction fine-tune of the Mistral AI 7B model and licensed under Apache 2.0, making it suitable for both commercial and non-commercial use.
The model excels in cybersecurity policies, programming, and assessing code vulnerabilities. However, I have not evaluated this model's safety. As such, you are encouraged to add your own alignment layer before deploying it as a service. Be aware that it may comply with any requests, including unethical ones. You bear responsibility for any content generated using this model. Use the model wisely.
Training
This model was trained using five datasets: Cyber-Logic, Code-Malade, Alpine-Ibex-Delta, Marmottes-Donnees, and Darknet-Diaries, all of which are available on my Hugging Face page. The model underwent training for 600 hours across 7.3 epochs using four 4090 GPUs.
Prompting
Format:
This model adheres to Mistral's instruction prompt format. Enclose your prompt within [INST]
and [/INST]
tokens. Start the first instruction with a begin-of-sentence ID, but subsequent instructions should omit this. The assistant's responses conclude with an end-of-sentence token ID.
<s>[INST] {prompt} [/INST]
This format can be utilized through the apply_chat_template()
method, which is available as a hugging face chat template.
Usage Example
import torch
from transformers import AutoModelForCausalLM, AutoTokenizer
model = AutoModelForCausalLM.from_pretrained("gussieIsASuccessfulWarlock/petit-lynx", torch_dtype=torch.float16)
tokenizer = AutoTokenizer.from_pretrained("gussieIsASuccessfulWarlock/petit-lynx")
device = "cuda" if torch.cuda.is_available() else "cpu"
model.to(device)
messages = [
{"role": "user", "content": "How do nation-state actors influence the cybersecurity environment, and what are the implications for global security?"},
]
encodeds = tokenizer.apply_chat_template(messages, return_tensors="pt")
model_inputs = encodeds.to(device)
model.to(device)
generated_ids = model.generate(model_inputs, max_new_tokens=1000, do_sample=True)
decoded = tokenizer.batch_decode(generated_ids)
print("\n\n")
print(decoded[0].split("[/INST]")[-1].replace("</s>", "").strip())
Example Outputs
- How do nation-state actors influence the cybersecurity environment, and what are the implications for global security?
Nation-state actors significantly shape the cybersecurity environment, primarily through cyber espionage, cyber warfare, and cyber propaganda. Here's a breakdown of their influence and the implications for global security:
1. Cyber Espionage: Nation-states often engage in cyber espionage to gain an economic or strategic advantage over other countries. This can involve stealing sensitive data such as trade secrets, research details, or intelligence information. The stolen data can be used to benefit the nation-state or to harm other countries' interests. This can lead to economic disruption, loss of competitive advantage, or diplomatic tension.
2. Cyber Warfare: Cyber warfare refers to attacks on computer systems, networks, or data with the intention of causing physical damage, disrupting critical infrastructure, or undermining national security. Cyber attacks can target a wide range of sectors, including energy, transportation, finance, and government. If successful, these attacks can lead to physical damage, loss of life, and significant economic loss.
3. Cyber Propaganda: Nation-states also use the cyber domain to spread propaganda and misinformation. This can be done through hacking and manipulating social media accounts, creating fake news websites, or launching targeted phishing campaigns. The goal is often to influence public opinion, sow discord, or undermine democracies.
The implications for global security are substantial:
1. Increased Tension and Conflict: Cyber attacks can escalate tensions between nations, potentially leading to military conflict. In response to a cyber attack, a nation-state may retaliate with additional cyber attacks, physical force, or even a combination of both.
2. Economic Instability: Cyber espionage and cyber attacks against critical infrastructure can lead to economic instability. For example, an attack on a nation's power grid could lead to widespread power outages, causing significant economic disruption.
3. Undermining Democracies: The spreading of misinformation and propaganda can undermine democracies by eroding public trust in institutions and manipulating public opinion. This can make it difficult for democracies to function effectively and make decisions in the best interests of their citizens.
4. Cyber Arms Race: As nation-states continue to invest in their cyber capabilities, there is a risk of a cyber arms race. This could lead to a future where cyber attacks are a normal and expected part of geopolitical competition, with nations spending vast amounts of resources to develop and deploy more advanced cyber weapons.
5. Cybersecurity Challenges for Businesses: Nation-state actors often target businesses in addition to governments. This can lead to increased cybersecurity challenges for businesses, as they must continually adapt to new threats and invest in cybersecurity measures to protect themselves.
In conclusion, nation-state actors play a significant role in shaping the cybersecurity environment, and their activities have wide-ranging implications for global security. It's crucial for international cooperation and the development of global cyber norms to help manage these risks. Additionally, businesses and individuals must also take cybersecurity seriously to protect themselves against nation-state cyber threats.
- I have a list of integers: [1, 3, -5, 2, -7, 9]. Could you help me find the largest sum of consecutive integers in this list?
To find the largest sum of consecutive integers, we'll need to iterate through the list and check the sum of adjacent integers. The algorithm I suggest will loop from the beginning to the end of the list and maintain the maximum sum found so far. Below is the Python code implementing this approach:
def largest_consecutive_sum(lst):
n = len(lst)
max_sum = lst[0] # Initialize with the first number
current_sum = lst[0] # Initialize with the first number as current sum
for i in range(1, n):
current_sum += lst[i]
if current_sum > max_sum:
max_sum = current_sum
if current_sum < lst[i]:
current_sum = lst[i]
return max_sum
# Test the function
numbers = [1, 3, -5, 2, -7, 9]
print(largest_consecutive_sum(numbers)) # Output: 12
In this example, there are two possible sums of consecutive integers that can be made from the list: `[1, 3]` and `[-5, 2, -7, 9]`. The function returns the maximum sum, which is `12`.
In case you have a negative number in the sum and want to exclude it, modify the code as follows:
# Function to find largest positive sum of consecutive integers
def largest_positive_consecutive_sum(lst):
n = len(lst)
max_sum = lst[0]
current_sum = lst[0]
for i in range(1, n):
if lst[i] > current_sum:
current_sum += lst[i]
elif lst[i] > 0:
current_sum = lst[i] # Reset current sum to the new positive number
if current_sum > max_sum:
max_sum = current_sum
return max_sum
# Test the function
numbers = [1, 3, -5, 2, -7, 9]
print(largest_positive_consecutive_sum(numbers)) # Output: 9
In this case, the output would be `9` since the largest positive sum is achieved with the series `[2, -7, 9]`, excluding the initial `[1, 3]` because `3` is not considered a positive number.
Training hyperparameters
The following hyperparameters were used during training:
- learning_rate: 5.e-6
- weight_decay: 0.01
- pct_start: 0.1
- seq_len: 8000
- batch_size: 1
- max_steps: 25500
- seed: 44
- distributed_type: multi-GPU
- num_devices: 4
- num_epochs: 7.3
- Downloads last month
- 0
Model tree for gussieIsASuccessfulWarlock/petit-lynx
Base model
mistralai/Mistral-7B-v0.3